# Application Interaction 1. [Why Applications Interact](./slides/why.md) 1. [Definitions and Terms](./slides/defs.md) 1. [App Classification](./slides/classification.md) 1. [Interaction Channels](./slides/channels.md) 1. [Roles of the Operating System](./slides/os-roles.md) 1. [Programming Interface](./slides/api.md) --- ## Firefox I/O ```console $ lsof -p $(pidof firefox) [...] firefox 32100 student 64u IPv4 38654330 0t0 TCP so:44750->lb-140-82-112-26-iad.github.com:https (ESTABLISHED) firefox 32100 student 65u IPv4 38659353 0t0 TCP so:37528->239.237.117.34.bc.googleusercontent.com:https (ESTABLISHED) [...] firefox 32100 student 176u unix 0x0000000000000000 0t0 38640969 type=SEQPACKET firefox 32100 student 178u unix 0x0000000000000000 0t0 38650545 type=SEQPACKET firefox 32100 student 179u unix 0x0000000000000000 0t0 38648379 type=STREAM firefox 32100 student 181r FIFO 0,13 0t0 38640924 pipe firefox 32100 student 185u a_inode 0,14 0 11438 [eventfd] firefox 32100 student 186uw REG 259,5 131072 17040026 /home/student/.mozilla/firefox/inonjd9q.default-release/protections.sqlite firefox 32100 student 188u unix 0x0000000000000000 0t0 38635209 type=STREAM [...] firefox 32100 student 200r REG 0,1 5196 14538955 /memfd:mozilla-ipc (deleted) [...] ``` ---- ### Reminder: I/O: Perspective of the Process - Communication / Interaction "outside" the process - "Inside" the process: working with memory, CPU instructions - "Outside" the process: I/O channels - File-like: data comes / goes from / to outside the system - Socket-like: data comes / goes from / to another process --- ## Why Do Applications Interact? - What is the alternative? - An application does everything. - Lack of modularity - Security / Reliability issues - Issues of maintainability - Modularity - Integration with existing components - Software reuse, but without building / linking --- ## Definitions and Terms --- - What does it mean that applications interact? - Do the following programs interact with other applications? --- ### Exhibit 1 ```c #include
int main(void) { printf("Hello, world\n"); return 0; } ``` ---- ### Exhibit 1 - The program simply prints a message to the standard output. - The terminal runs the program, so we do have some app interaction. - Also, it can interact with other programs by doing: `./hello_world | grep Hello` - Interaction is static. - However, this is not a voluntary interaction on the part of the program. - The program was designed as a standalone one. ---- ### Exhibit 1 - Creating Interaction - `demo/send-receive/reader.c` - `demo/send-receive/writer.c` - `demo/send-receive/send_receive_pipe.c` --- ### Exhibit 2 ```c // writer.c int main(void) { FILE *fp = fopen("myf.txt", "r"); fprintf(fp, "Hello"); fclose(fp); return 0; } ``` ```c // reader.c int main(void) { char a[20]; FILE *fp = fopen("myf.txt", "r"); fscanf(fp, "%s", a); printf("%s\n", a); fclose(fp); return 0; } ``` ---- ### Exhibit 2 - The 2 programs indeed interact. - But is there any difference from the previous example? - There is no protocol of interaction. - If one of the applications did not exist, the other one could still be a valid, meaningful program. ---- ### Exhibit 2 - What if we start the reader before the writer? - Synchronization is required. ---- ### App Interaction - Communication (data transfer) - Synchronization (notification) --- ### Exhibit 3 ```c int main(void) { pid_t pid = fork(); if (pid == 0) { sleep(2); exit(EXIT_SUCCESS); } waitpid(pid, &status, 0); return 0; } ``` ---- ### Exhibit 3 - Synchronization, but no communication - The programs interact in a structured manner. - One process waits for the other to finish. - Interaction is dynamic - e.g. a process actively waits for the other. - The 2 processes have been designed to work together. --- ### Exhibit 4 - WhatsApp Application  ---- ### Exhibit 4 - WhatsApp Application - There is interaction between the main client application and other applications: photos, location, YouTube etc. - There is also interaction with the web server when sending messages. --- ### App Interaction - Applications that run at the same time and that communicate with each other during their lifetimes. - This communication involves: - passing messages (**communication**) - sending / waiting for notifications (**synchronization**) ---- ### Requirements for Interaction - Address / Identity of processes - Process ID - Hostname, port - Communication channel - Buffer to store data - "Endpoints" to send / receive data from buffer - Address / Identity of channel - Path in filesystem - Hostname, port, path - ID ---- ### Metrics and Goals for Interaction - Speed: fast transfer - Latency: time to actual delivery - Reliability - Intra-system interaction - Security: access control - Inter-system interaction - Security: confidential connection - Scalability: multiple connections --- ## App Classification --- ### App Components - Threads and processes - Processes may be colocated on the same system - Processes may be distributed on multiple systems ---- ### App Components  --- ### App Classification - Single-process single-threaded - Multi-threaded - Multi-process - Multi-system - These apps use OS primitives to communicate between components (threads, processes) ---- ### Single-process Single-threaded - No actual software component interaction - Simple applications that don't rely on complex features - `ldd
| grep pthread` - `/bin/bash`, `/bin/dd` ---- ### Single-process Multi-threaded Homogeneous - Multiple threads doing the same work - Threading models: boss-workers, worker threads, thread pools - Generally little interaction: join at the end - multi-threaded web servers - `libx264` library for `ffmpeg` - Firefox web browser: browser tabs ---- ### Single-process Multi-threaded Heterogeneous - Multiple threads, each doing different work - Firefox web browser: browser management processes ---- ### Multi-process Homogeneous - Multiple processes doing the same work - Process pool - Generally no interaction: pre-fork, get job, serve - Multi-process web servers (Apache2 mpm-prefork) - Google Chrome: a process per Tab ---- ### Multi-process Heterogeneous - Multiple processes doing different items - IPC mechanisms (pipes, message queues, sockets) to interface between processes - Postfix - GitLab ---- ### Postfix  ([source](https://commons.wikimedia.org/wiki/File:Postfix_architecture.svg)) ---- ### GitLab
([source](https://docs.gitlab.com/ee/development/architecture.html)) ---- ### Multi-system - Processes run on different systems - Used in distributed systems, data centers, computing clusters - Typically a combination of homogeneous and heterogeneous systems and processes - Kubernetes, OpenStack, Netflix backend servers ---- ### Kubernetes 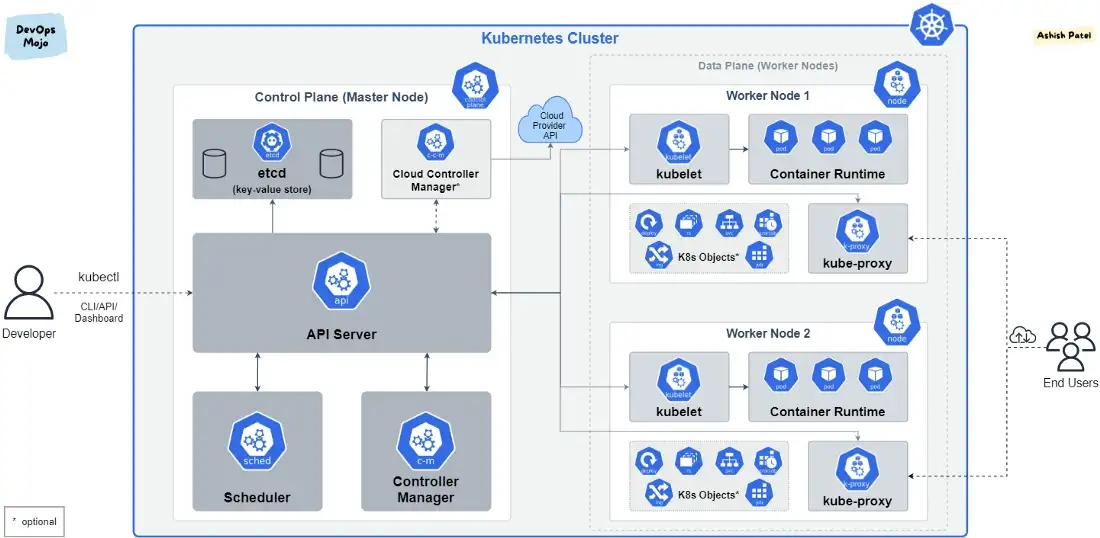 ([source](https://medium.com/devops-mojo/kubernetes-architecture-overview-introduction-to-k8s-architecture-and-understanding-k8s-cluster-components-90e11eb34ccd)) --- ## Interaction Channels --- App components interact via interaction channels. ---- ### Interaction Types - Sending / waiting for notifications (**synchronization**) - Passing messages (**communication**) ---- ### Reminder: App Components - Threads and processes - Processes may be colocated on the same system - Processes may be distributed on multiple systems ---- ### Interaction Use-Cases - Inter-thread interaction (the same process) - Inter-process colocated - Inter-process distributed --- ### Synchronization - One component waits, the other notifies - A synchronization object is required - The object typically has a binary value: set or unset - Notification may happen without a wait - Interruption of normal execution flow ---- ### Synchronization 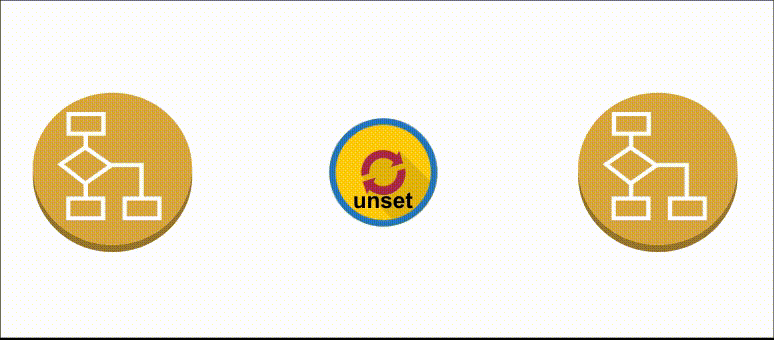 ---- ### Interruption 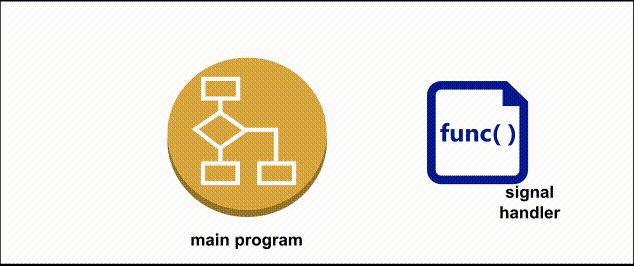 --- ### Communication - One component sends / writes data, the other receives / reads it - A communication buffer is required - Accessing the buffer - Directly, via memory address: shared memory - Indirectly, via endpoints (file descriptors) - Required for distributed communication ---- ### Shared Memory - Threads share the virtual address space of a process - Different threads use the same virtual address - Processes use virtual memory to share a phyisical memory region - Different processes use different virtual addresses mapped to the same physical address - Communication is not synchronized - Receiver doesn't know when data has been sent ---- ### Shared Memory  ---- ### Communication Channel - Underlying buffer is not directly exposed - Access via endpoints (file descriptors) - The only mean for distributed communication - For distributed communication, there are multiple buffers: one on each host, buffers in middleboxes - Synchronization is implicit - Reader is blocked (waits) until data is available - Writer is blocked (waits) until room is available - Protocols are used to make communication reliable ---- ### Communication Channel  --- ## Roles of the Operating System --- ### Reminder: Requirements - Address / Identity of processes - Process ID - Hostname, port - Communication channel - Buffer to store data - "Endpoints" to send / receive data from buffer - Address / Identity of channel - Path in filesystem - Hostname, port, path - ID --- ## OS Roles - Operating system mediates app interaction - Operating system provides primitives / mechanisms for app interaction - Roles - registry: identifying app endpoints - post office: reliably passing messages between applications - police: ensuring access control and security - doorbell: app notification of incoming messages ---- ## OS - The Lawmaker - The OS is like a lawmaker the dictates how the interaction takes place - Allows or denies the sending of data / notifications - Ensures correct delivery of data / notification ---- ## OS - The Lawmaker  --- ## Programming Interface --- ### Summary of Channel Types - Synchronization - Interruption - Shared memory - Communication channels --- ### Synchronization - Synchronization object - To be created / allocated before use - mutex / spinlock / file locking: `lock()` / `unlock()` - semaphore: `up()` / `down()` - monitor / condition variable: `wait()` / `notify()` - signals (Unix / Linux): `kill()` / `sigwait()` - Generally used for inter-thread synchronization ---- ### Inter-Process Synchronization - Named semaphores - Entry in filesystem - File locking - Uses actual file ---- ### Synchronization Demos In `./demo/lock/` In `./demo/sync/` --- ### Interruption - Asynchronous to normal execution flow - Generally used for inter-process interaction on the same system - Associate function handler to run when interruption is received - signals (Unix / Linux): `sigaction()`, `kill()`, `sigqueue()`, `sigsuspend()` - exceptions (Windows): `AddVectoredExceptionHandler()`, `RaiseException()` --- ### Interruption Demos In `./demo/interrupt/` --- ### Shared Memory - Implicit for inter-thread communication - Explicit API for processes - Create shared memory mapping - Filesystem entry as backing store - No API for using it - Just use memory operations ---- ### Shared Memory Demos In `./demo/shared-mem/` --- ### Communication Channels - Discussed in I/O chapter, socket-like - Created before-hand - `read()` / `write()`, `send()` / `recv()` - (anonymous) pipes: related processes - named pipes (filesystem entry): any colocated processes - UNIX / local sockets (filesystem entry): any colocated processes - network sockets: colocated or distributed processes ---- ### Communication Channels In `./demo/comm-channels/` ---- ### Case study: Our Own Server - Server computes Fibonacci, sends it back - single-process server - multi-threaded server: uses a thread per-connection - multi-process server: use a process per-connection - thread-pool server: uses pre-created threads to serve - process-pool server: use pre-created processes to serve ---- ## Case study: Our Own Server In `./demo/fibonacci-server/`